So far, I have been playing around with controller driven UE4 connections, where the Arduino acts as a platform to control elements within UE4. I’m also interested in using UE4 interactables to drive physical electronics, as this might be useful when trying to sync up physical and virtual camera settings. A simple Arduino Servo tutorial in the starter kit helped me think about triggering a servo from an in-game
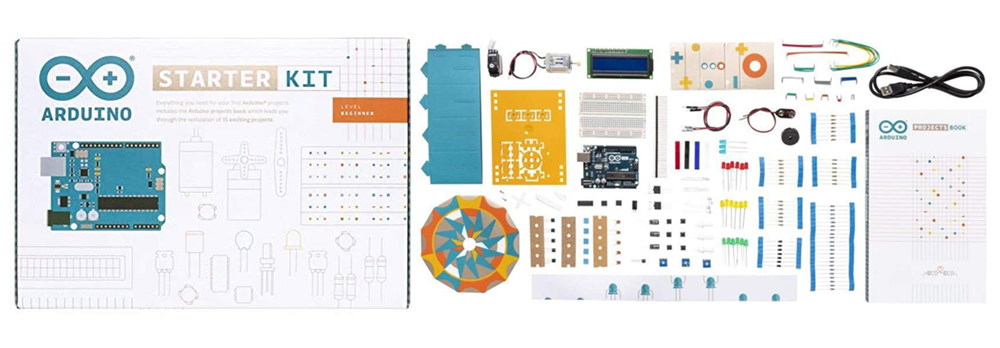
If you are new to the Arduino like I am, I highly recommend the starter kit listed above (it’s an Amazon link, so go buy it!!). The kit comes with the Arduno Uno, a breadboard so you can link up your components, a wide range of components and sensors you can start to play with, and most importantly a little project book which contains a wide range of fun little projects and instructions. Many of the projects I am playing with stem from basic example projects, and thinking what I can do to use them in UE4.
The project I started with was the ‘Mood Cue’ – you can find the project on the PDF version of the Projects Book found here on page 63. The base code and circuit diagram can be found there, so I won’t specifically go into details on that. The project essentially controls a Servo using the Servo library, and a potentiometer to turn the Servo. The project decorates this with cardboard cutouts, however I had no interest in actually creating the project, only to understand how sensors and servos actually work with the Arduino. Many of my projects will be mainly learning from the sample projects, and identifying useful elements for projects connected to virtual cinematography. This is the result of the ‘Mood Cue’ project.
From this, I wanted to see if I could drive the Servo from events within UE4. The thought process was we can control virtual cameras within UE4 via physical interfaces and Potentiometers/Buttons/Sensors, but can we have UE4 control a real camera? Part of my Masters will involve having virtual interactable environments which respond to text input commands (if I can figure out how to do it!). It would be good to have UE4 control the position, focus, camera zoom as well in some situations (sliders and motors for example).
The UE4 setup – Event driven Servo triggers
The scene is quite simple, three trigger boxes set up across a plane. Each of those trigger boxes sends a command to the Arduino to trigger an if statement within the main operational loop. The specific if statement triggered is determined by the number specified within the EventOverlap function, so only the triggered instruction within engine will alter the Servo position. First box sets the angle of the Servo to 0 degrees, second 90 degrees, and third sets the servo to 179 degrees (max). The final setup requires no Potentiometer, however the videos still show them on the breadboard from the original Mood Cue project.
This is a screenshot of the setup within UE4, very simple:

This is a screenshot of the EventOverlap graphs within UE4:
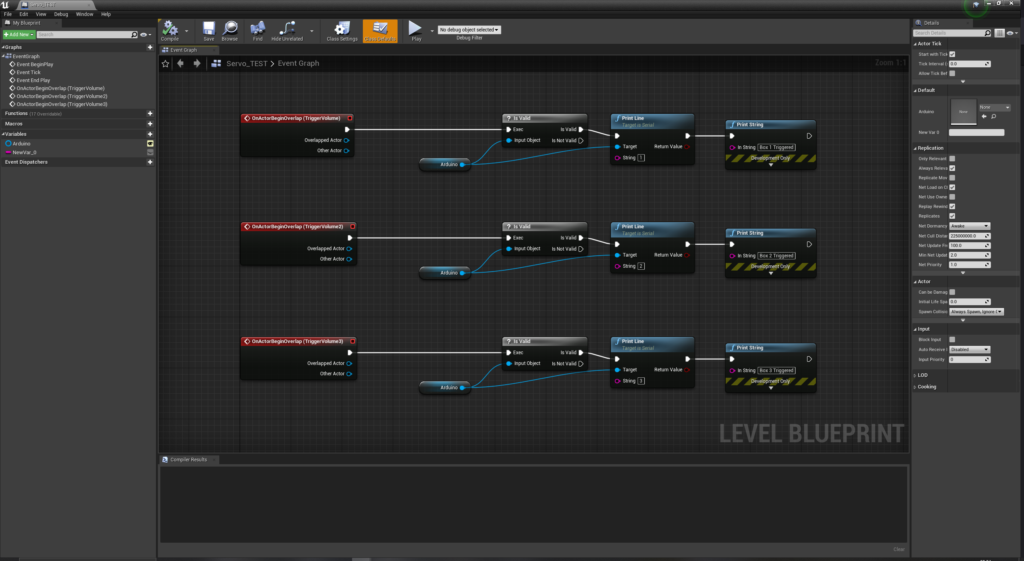
This is a video of it working:
Here is the code:
#include <Servo.h>
Servo myServo;
void setup() {
// put your setup code here, to run once:
myServo.attach(9);
Serial.begin(9600);
}
void loop() {
// put your main code here, to run repeatedly:
if (!Serial.available()) return;
while(Serial.available()){
char str = Serial.read();
if (str == '1'){
myServo.write(0);
}
if (str == '2'){
myServo.write(90);
}
if (str == '3'){
myServo.write(179);
}
}
delay(15);
}
Conclusion:
While this project isn’t very complex, it does provide guidance as to how events can trigger specific triggers on the Arduino. You could imagine using this to turn on lights, motors, start timers, servos, any component you want to set up on your Arduino. The basics are being able to provide stable, accessible and responsive inputs into UE4, and also the opposite direction with UE4 providing immediate instructions to trigger events on the Arduino. Hopefully, this will be useful for future projects moving forward. My next stage will be to push forward with other Arduino projects, and play with different sensors and components with the goal to identify what will be useful moving forward.